Comprehensive Guide To Java
Introduction
Java is one of the most popular programming languages globally, known for its versatility, platform independence, and robustness. This guide provides a deep dive into Java programming, covering essential concepts and tools that enable developers to build everything from small applications to large-scale enterprise systems. It emphasizes Object-Oriented Programming (OOP) principles, Java frameworks, and best practices to help both beginners and experienced developers become proficient in Java.
The guide also explores advanced Java EE (Java Enterprise Edition) concepts and frameworks such as Hibernate and Spring, which are critical for building scalable, high-performance enterprise applications. Whether you're new to Java or looking to expand your skills, this guide provides the foundation and advanced knowledge needed to succeed in a Java-based development environment.
Table of Contents
- Introduction to Java
- Object-Oriented Programming (OOP)
- Java Collections Framework
- Exception Handling
- Java Enterprise Essentials
- JDBC, Servlets, and JSP
- Hibernate and ORM (Object-Relational Mapping)
- Spring Framework
- Spring Boot
- Microservices with Java
Java Programming Concepts
Introduction to Java
Java is a high-level, object-oriented programming language designed to be platform-independent. With the Java Virtual Machine (JVM), Java code can run on any system with the JVM installed, providing "write once, run anywhere" capability. This section covers the fundamentals of Java syntax, data types, operators, and control structures. It provides a foundational understanding of Java programming, helping beginners get started with core concepts like classes, objects, methods, and packages.
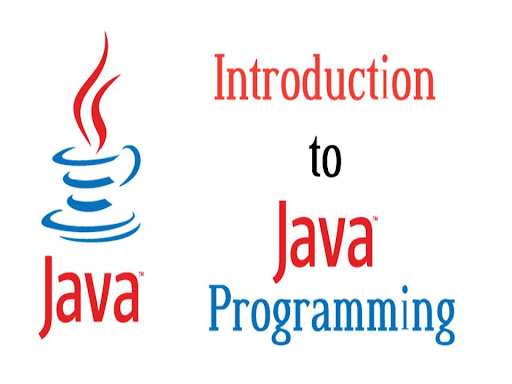
Object-Oriented Programming (OOP)
Java is fundamentally an object-oriented programming language, which means it leverages the four principles of OOP: Encapsulation, Abstraction, Inheritance, and Polymorphism. This section introduces OOP principles and demonstrates how to implement them in Java. By understanding these concepts, developers can create modular, reusable, and organized code structures, improving the maintainability and scalability of applications.
Java Collections Framework
The Java Collections Framework provides a set of data structures and algorithms to store, retrieve, and manipulate collections of data. This includes classes like ArrayList, HashMap, LinkedList, and HashSet. Mastering these classes allows developers to handle complex data operations efficiently, which is crucial for building scalable applications. This section covers collections in detail, from basic usage to more advanced features like sorting, filtering, and concurrency.
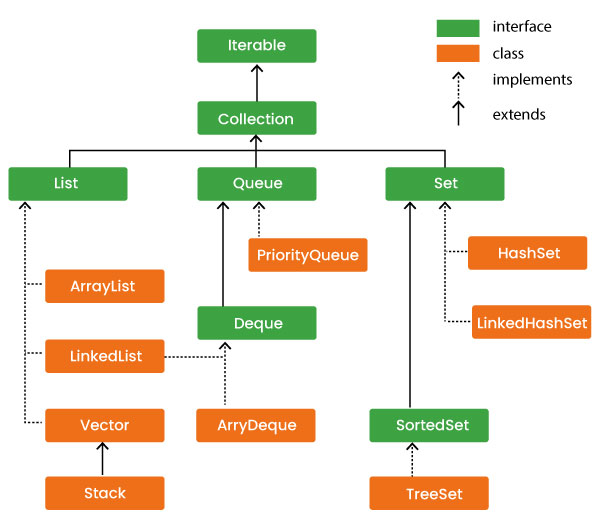
Exception Handling
Exception handling is a critical aspect of Java programming, enabling developers to manage runtime errors gracefully. This section covers the basics of try-catch blocks, throws, throw, and finally. Understanding how to handle exceptions properly helps in creating robust and fault-tolerant applications. Developers will learn best practices for managing checked and unchecked exceptions, ensuring code reliability and stability.
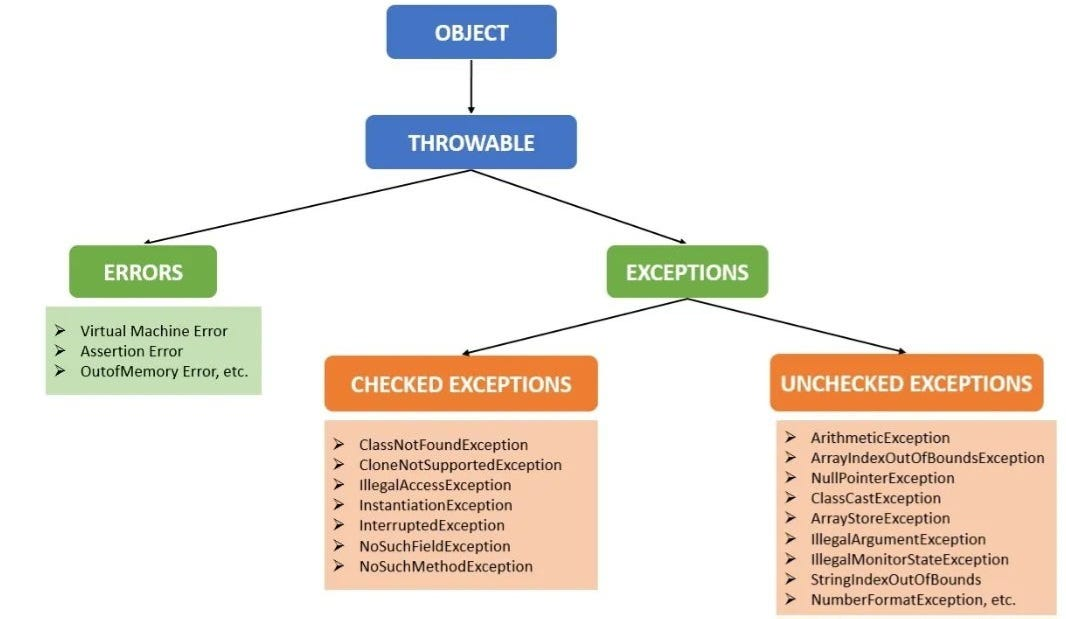
Java Enterprise Essentials
Java EE (Java Enterprise Edition) provides a platform for building large-scale, distributed, and multi-tiered enterprise applications. This section introduces essential concepts in Java EE, such as dependency injection, EJB (Enterprise JavaBeans), and JNDI (Java Naming and Directory Interface). It prepares developers to work in enterprise environments where Java EE applications are widely used for business-critical systems.
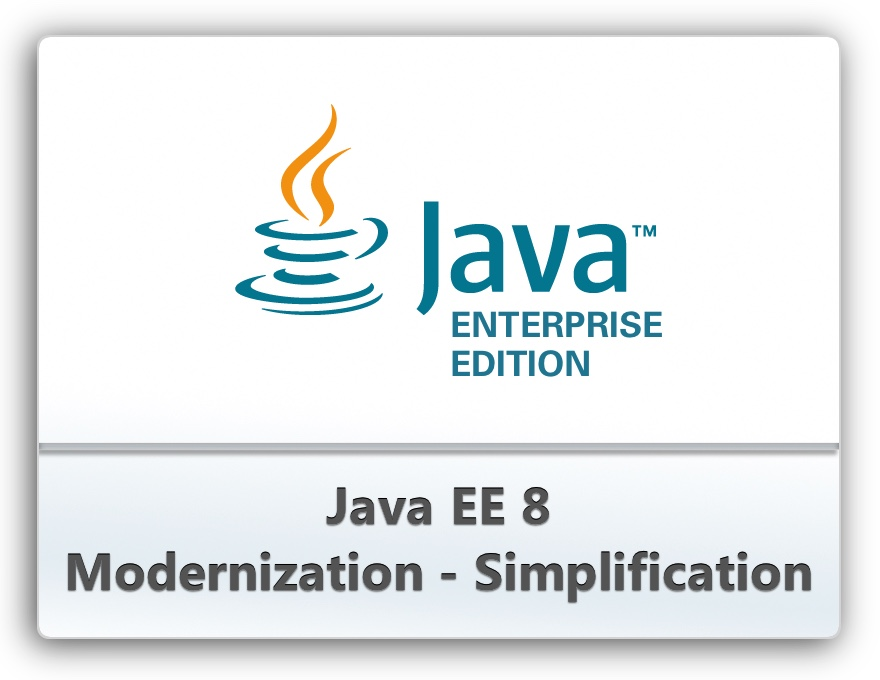
JDBC, Servlets, and JSP
JDBC (Java Database Connectivity), Servlets, and JSP (JavaServer Pages) are fundamental for building web applications in Java. JDBC allows Java applications to interact with databases, enabling CRUD (Create, Read, Update, Delete) operations. Servlets and JSP facilitate dynamic content generation for web applications. This section covers how to connect to databases, build backend logic, and generate dynamic HTML pages using Java, laying the groundwork for full-stack Java development.
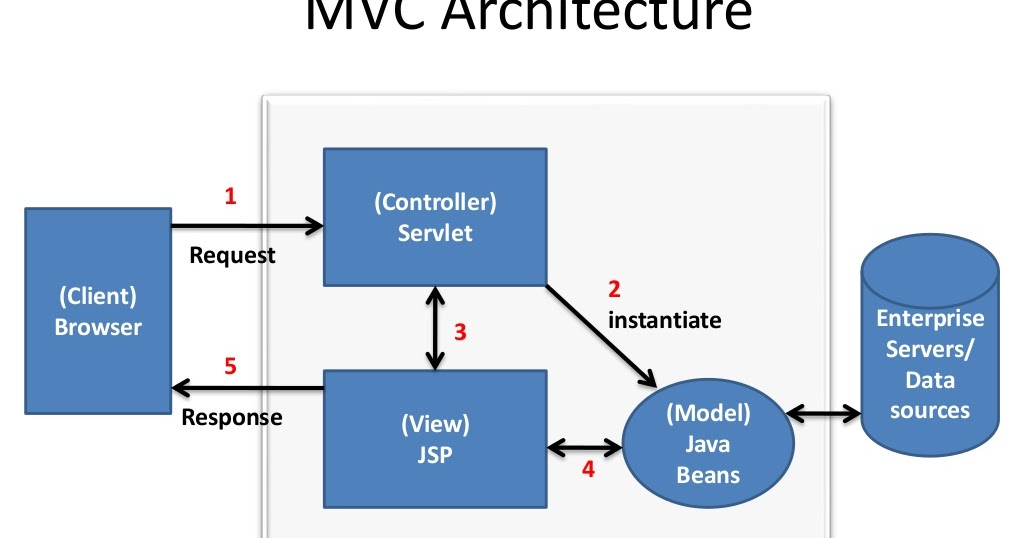
Hibernate and ORM (Object-Relational Mapping)
Hibernate is a popular ORM (Object-Relational Mapping) framework that simplifies database operations by mapping Java classes to database tables. This section introduces Hibernate basics, including mapping entities, CRUD operations, and querying databases using HQL (Hibernate Query Language). Hibernate eliminates the need for complex SQL queries, allowing developers to interact with databases in a more object-oriented manner. Understanding Hibernate is crucial for building data-driven Java applications efficiently.
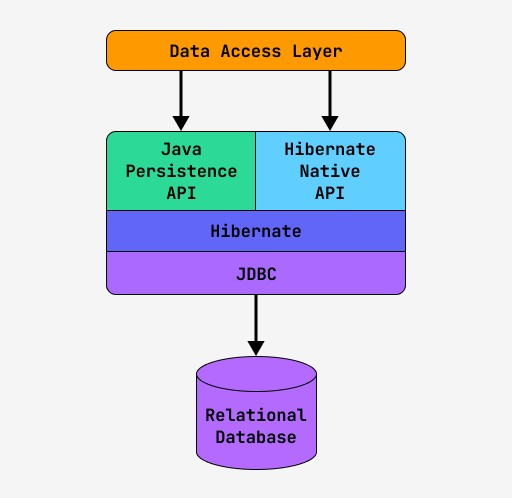
Spring Framework
The Spring Framework is a comprehensive framework for building enterprise Java applications. It provides tools for dependency injection, transaction management, and aspect-oriented programming (AOP), making it easier to create loosely coupled, maintainable code. This section covers core Spring components such as Spring Core, Spring MVC, and Spring Data JPA, preparing developers to build modular, scalable applications with Spring.
Spring Boot
Spring Boot is an extension of the Spring Framework that simplifies the setup and development of production-ready applications. With its opinionated approach, Spring Boot minimizes the need for boilerplate configuration, allowing developers to focus on application logic. This section covers building RESTful APIs, configuring Spring Boot, and deploying Spring Boot applications, making it an essential tool for rapid application development in Java.
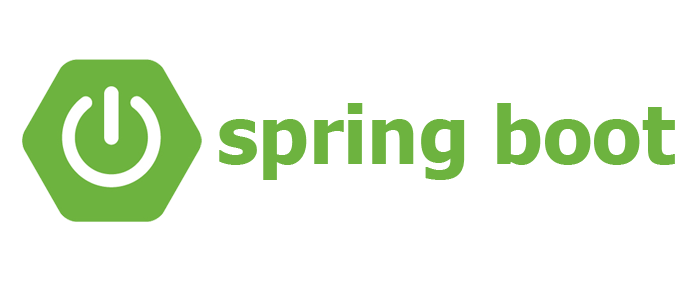
Microservices with Java
Microservices is an architectural style where applications are composed of small, loosely coupled services that can be independently deployed and scaled. This section explores building microservices using Spring Boot and Spring Cloud, covering topics like service discovery, load balancing, and API Gateway. Microservices are essential for modern application development, and Java, combined with Spring Boot, provides a robust framework for implementing them.
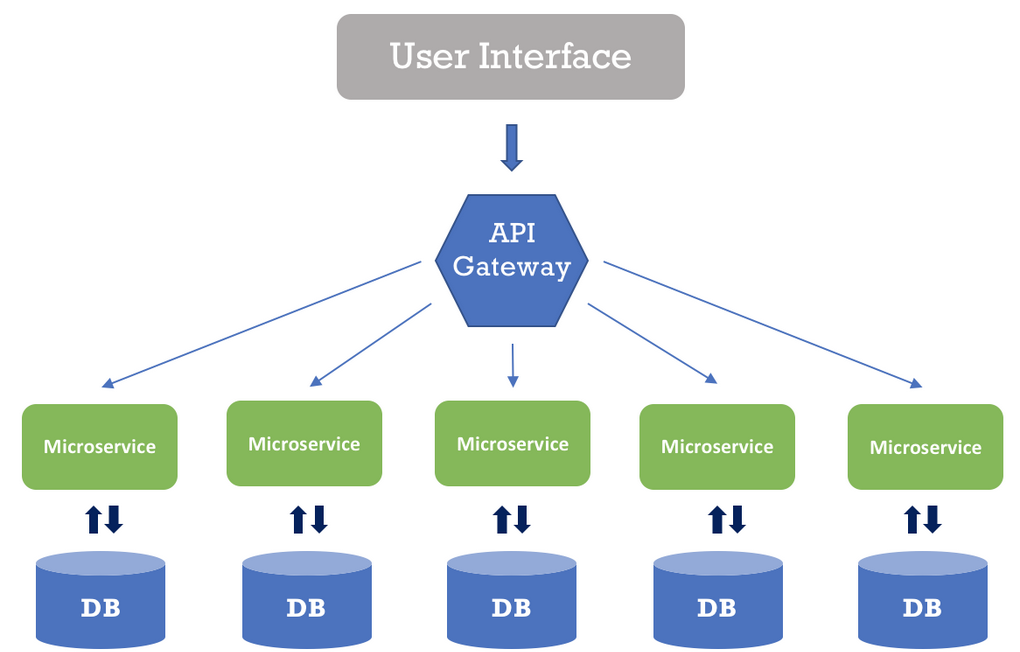
Conclusion
ava remains a vital language in the software industry due to its platform independence, performance, and versatility. From web applications to Android apps, Java's rich ecosystem supports a wide range of applications. For beginners, mastering core concepts like OOP, Collections, and Exception Handling builds a solid foundation. For advanced developers, understanding frameworks like Spring, Hibernate, and microservices architecture enables the development of robust and scalable enterprise applications.
PROJECTS
JOB ROLES
Java Developer
A Java Developer is responsible for writing, testing, and maintaining Java applications. They work on building applications using core Java concepts and frameworks, including Java SE (Standard Edition) and Java EE (Enterprise Edition). Java Developers are skilled in OOP principles, data structures, and algorithms, often working on backend systems and integrating with databases and other services.
Fullstack Developer
A Fullstack Developer proficient in Java is capable of working on both frontend and backend development. They use Java for backend development, often combining it with frontend technologies like HTML, CSS, JavaScript, and frameworks like Angular or React. They are skilled in building end-to-end solutions, handling everything from database management to UI development.
Java EE Developer
A Java EE Developer specializes in building enterprise applications using Java EE technologies, including Servlets, JSP, EJB, JPA, and Web Services. They design and implement scalable, secure, and transaction-based applications, often working in financial, healthcare, and e-commerce domains. Java EE Developers focus on backend and middleware components that handle complex business logic and large data operations.
Java Android Developer
A Java Android Developer is responsible for developing mobile applications for the Android platform using Java. They work with Android SDK, XML, SQLite, and other tools specific to Android development. Java Android Developers are skilled in building user interfaces, handling mobile-specific challenges, and integrating with backend services to provide a seamless mobile experience.
Software Developer
A Software Developer with a focus on Java may work across various domains, creating desktop, web, or mobile applications. They use Java along with other tools and languages as required, adapting their skills to different project needs. Software Developers write, test, and maintain software, often collaborating with other team members to deliver high-quality solutions.
RESUME
Ravi Krishna
E-mail: ravi2krishna@gmail.com
Mobile: +91 9999999999
Linkedin: https://in.linkedin.com/in/ravi-krishna-5680b330
GitHub: https://github.com/ravi2krishna
Objective
Java Developer with 3 years of experience in developing, testing, and deploying enterprise-level applications. Skilled in designing scalable, efficient, and secure solutions using Java technologies and frameworks such as Spring, Hibernate, and RESTful web services. Proficient in Agile methodologies and experienced with CI/CD practices to streamline software development processes.
Professional Summary
- 3 Years of experience in Java software development, specializing in backend development and enterprise applications.
- Expertise in Java SE/EE, Spring Framework (Spring Boot, Spring MVC), Hibernate, and RESTful web services to build robust applications.
- Proficient in Microservices architecture, using Spring Boot and REST APIs to develop scalable and maintainable services.
- Experience with front-end integration using JavaScript frameworks like Angular and React for end-to-end development.
- Knowledgeable in database management with MySQL, PostgreSQL, and Oracle; skilled in writing complex SQL queries and database optimization.
- CI/CD experience using Jenkins, GitHub Actions, and Git for automating the deployment process.
- Testing and Quality Assurance expertise using JUnit, Mockito, and TestNG to ensure code quality and reliability.
- Familiar with containerization and deployment using Docker and Kubernetes for efficient application scaling.
- Skilled in collaborating with cross-functional teams to deliver high-quality solutions that meet client requirements.
Skills Profile
- Programming Languages: Java, SQL, JavaScript
- Frameworks: Spring Boot, Spring MVC, Hibernate, JPA
- Web Services: RESTful APIs, SOAP
- Database Management: MySQL, PostgreSQL, Oracle
- Tools and Platforms: Docker, Jenkins, Git, GitHub, Eclipse, IntelliJ IDEA
- Testing: JUnit, Mockito, TestNG
- Version Control: Git, GitHub, GitLab
- Methodologies: Agile (Scrum), Test-Driven Development (TDD)
- Front-end Technologies: HTML, CSS, JavaScript, Angular, React
Certifications
- Oracle Certified Professional, Java SE 11 Developer – 2022
- Spring Professional Certification – 2023
Experience
Java Developer | XYZ Tech Solutions | Hyderabad, Telangana (India)
Customer: Global Bank, FinTech Inc.
Role: Java Developer (Spring Boot, REST APIs, Microservices)
Period: March 2023 – Present
Roles and Responsibilities:
- Developed and maintained microservices using Spring Boot, enhancing system scalability and performance.
- Designed and implemented RESTful APIs to facilitate communication between client applications and backend systems.
- Integrated microservices with databases like MySQL and PostgreSQL, optimizing queries for improved data retrieval times.
- Collaborated with front-end developers to integrate back-end Java services with Angular-based user interfaces.
- Configured CI/CD pipelines using Jenkins to automate code deployment and reduce release times.
- Utilized Docker and Kubernetes to containerize applications and orchestrate deployments across environments.
- Employed JUnit and Mockito for unit testing, ensuring high code quality and reliability.
- Monitored application performance and implemented optimizations to reduce system latency and improve user experience.
Java Developer | ABC Digital Solutions | Bangalore, Karnataka (India)
Customer: E-Commerce Ltd., SaaS Solutions Inc.
Role: Java Developer (Java EE, Spring, Hibernate)
Period: Feb 2021 – Feb 2023
Roles and Responsibilities:
- Designed and developed backend services using Java EE, Spring MVC, and Hibernate for various e-commerce and SaaS applications.
- Created and maintained complex SQL queries and procedures in MySQL for data retrieval and transaction management.
- Developed RESTful web services to facilitate seamless integration with client applications and third-party services.
- Implemented caching mechanisms to enhance application performance and reduce database load.
- Built custom exception handling and logging frameworks for better monitoring and error tracking.
- Worked closely with product and design teams to understand requirements and translate them into technical specifications.
- Assisted in maintaining code quality and security standards by following best practices and conducting regular code reviews.
Junior Java Developer | DEF Tech Services | Hyderabad, Telangana (India)
Customer: Start-Up X
Role: Junior Java Developer
Period: Dec 2020 – Jan 2021
Roles and Responsibilities:
- Assisted senior developers in designing Java-based backend components for web applications.
- Participated in the development of CRUD operations using Java and Hibernate.
- Wrote unit tests with JUnit to ensure functional accuracy of code modules.
- Supported front-end team by integrating API endpoints with client-side applications.
- Gained experience in Agile development practices and contributed to daily stand-ups and sprint planning sessions.
Educational Background
- Bachelor of Technology in Computer Science Engineering – Osmania University – 2019
- 12th Standard – CBSE Board – 2015
- 10th Standard – Telangana State Board – 2013
This format provides a concise and professional overview suitable for a Java Developer position. Adjust any specific details as needed for accuracy.